I did something almost identical in Retool Mobile recently and it was very easy, since in Mobile you can assign data sets to a list view, which makes looping through the records of a data set a breeze.
Conversely, in Web Apps, you have to work off the main query and keep track of ri's and i's which can get confusing.
In your scenario, although seemingly simple, there is a fundamental issue with the data structure, which adds unnecessary complexity to everything.
Typically, in an actual application, you would likely not have the data structured that way in the database. Instead, tickets would be in their own table with their own property columns (ticket id, ticket status, ticket category, etc.)
Then, in a separate table, you'd have a list of tasks, associated with a ticket id. It is here where your scenario starts. The difference is you're missing a step, and that is the initial select of the unique ticket id, through which you would loop and request the associated tasks from the tasks table.
So first you'd have something like: "Select * from ticketsTable where ticketStatus = active", and then for every row/ticket id from that query, you'd loop to get the tasks: "Select * from tasksTable where ticket_id = ... "
The first order of things is to get your data structured as above. For this, in Retool, you don't need multiple queries like you mentioned. You need only a basic one, and then some further processing to transform the query results in the right format (using a query transformer).
The main (and only) query is:
select * from ticketsTable;
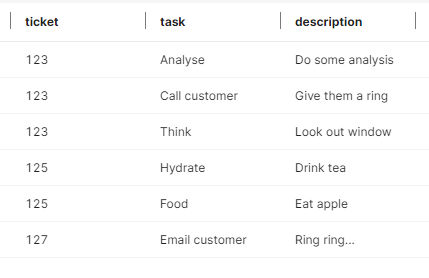
Then, on the same query, enable a transformer:
//determine the number of unique tickets (using underscore library function)
const ticketsArray = _.uniq(data.ticket); //e.g. output: [123,125,127]
//initialize an empty array
let ticketsObj = [];
//loop through the array of unique tickets
for (const ticket of ticketsArray){
//get the ticket tasks by filtering the sql query output
let ticketTasks = formatDataAsArray(data).filter(tasks => tasks.ticket == ticket);
//add ticket number and its tasks to tickets object
ticketsObj.push(
{
ticket_id: ticket,
tasks: ticketTasks
}
)
}
return ticketsObj;
The above transforms the query output in this format:
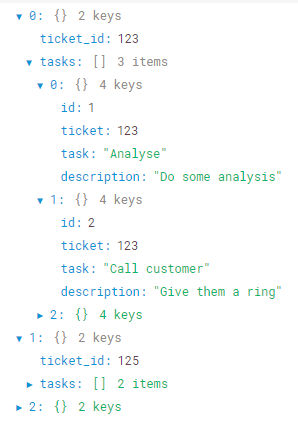
With the data in this format (based off a single getTickets query), the rest follows nicely:
There is also some useful information in this docs article: https://docs.retool.com/docs/create-custom-list-views