Hello,
I'm using array.find to find the object in a temporary state array that matches a given packinglistno and packno. I'm doing this from a JS Query.
After finding a match, I want to change the value of property "scanned" for that specific object to true.'
var packToUpdate = packsArray.value.find(packItem => packItem.pack === pack)
if (packToUpdate && packsArray.value['0'].packinglist === packinglist) {
// packinglist and packno match and not scanned yet - update pack to true
packToUpdate.scanned = true
console.log(`Updated scanned value for pack ${pack}`)
} else if (packToUpdate && packToUpdate.packinglist === packinglist && packToUpdate.scanned === true) {
// match, but already scanned
console.log('Already scanned!')
} else if (packToUpdate && packToUpdate.packinglist != packinglist) {
// pack match but packinglist doesn't match SHOW POPUP
console.log('Wrong packinglist no!')
} else {
// no match packinglist and pack
console.log(`Could not find pack with packlist ${packinglist} and pack ${pack}`)
}
console.log(packsArray.value)
packToUpdate.scanned = true does show it as updated in the console, but the state itself doesn't get changed.
I assume I'll have to use SetIn, but I don't know how to write this.
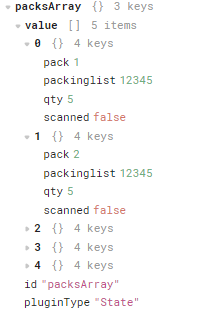
Any help would be appreciated. Thanks
That's correct, the setIn function is what you'll want to use.
It accepts arguments for the index, the property and the value eg
packsArray.setIn([1, 'scanned'], false);
This would set the "scanned" property of the object at index 1 (ie second record) in the array to false;
Thanks, that seems to do the trick.
I've replaced the static index '1' from you example with the index that is being returned by the line below.
const packToUpdate = packsArray.value.findIndex(packItem => packItem.pack === pack)
packsArray.setIn([packToUpdate, 'scanned'], true)
For some reason, this is working perfectly fine for all the packs/objects, but not for pack no '1'.
The index returned is 0, so i don't understand why it doesn't work.
that's strange, I can't replicate that issue locally. Maybe need to take a look at the code/data and see if we can figure out why it's not happy with index 0.
option_list.setIn([option_list.value.findIndex(i => i.value === 1), "label"], "a")
Thanks for helping me out. Will try again if I'm able to get my app back. I''ve lost all my progress from yesterday.
I've found the issue, it was in the evaluation of the condition:
if (packToUpdate && packsArray.value['0'].packinglist === packinglist) {
packTo Update returns 0 for the first element of the array.
The evaluation in the if condition checks for packToUpdate equals True. 0 evaluates as False, so it didn't meet the condition criteria.
Changing the conditon to this solved it:
if (packToUpdate >= 0 && packsArray.value['0'].packinglist === packinglist) {
Element not found will result in packToUpdate to be -1 instead of False.
Hi @mbruijnpff!
setIn definitely sounds like a correct approach. Would something like this work? If not, please do let me know and I'd be happy to debug further with you 
var packToUpdate = packsArray.value.find(packItem => packItem.pack === pack)
if (packToUpdate && packsArray.value['0'].packinglist === packinglist) {
// packinglist and packno match and not scanned yet - update pack to true
var updatedPackToUpdate = setIn(packToUpdate, ['scanned'], true)
var updatedPacksArray = setIn(packsArray, ['value', packsArray.value.indexOf(packToUpdate)], updatedPackToUpdate)
console.log(`Updated scanned value for pack ${pack}`)
} else if (packToUpdate && packToUpdate.packinglist === packinglist && packToUpdate.scanned === true) {
// match, but already scanned
console.log('Already scanned!')
} else if (packToUpdate && packToUpdate.packinglist != packinglist) {
// pack match but packinglist doesn't match SHOW POPUP
console.log('Wrong packinglist no!')
} else {
// no match packinglist and pack
console.log(`Could not find pack with packlist ${packinglist} and pack ${pack}`)
}
console.log(updatedPacksArray)