Tom_I
1
Hi All, I am trying to put a delay in the below query so that it avoids a rate limit. How do I go about this?
const arr = (table1.data)
const query = stripeCustGetEmail
const promises = arr.map((item) => {
return query.trigger({
additionalScope: {
assignment_id: item.email
}
});
});
return Promise.all(promises)
You can try something like this. I verified this with ChatGpt, and it looks good, but check your requirements before using it.
const arr = table1.data;
const delay = 1000; // milliseconds between calls (adjust as needed)
async function getEmailWithDelay(email) {
try {
await new Promise(resolve => setTimeout(resolve, delay));
return query.trigger({
additionalScope: {
assignment_id: email
}
});
} catch (error) {
// Handle errors here
}
}
const promises = arr.map(async (item) => {
return await getEmailWithDelay(item.email);
});
return Promise.all(promises);
You can also set the "Run this query periodically" property.
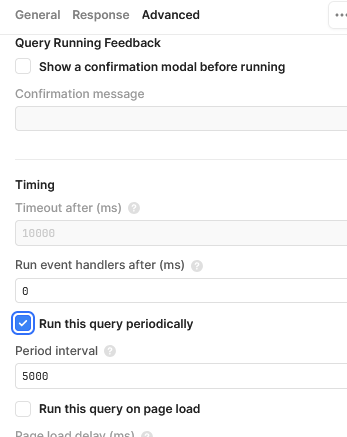