First array GetLocations.data
:
[
{
"id": 1,
"uid": null,
"name": "GLACO",
"prefix": "GLA",
"description": "Glasgow CO",
"latitude": "39.22681032",
"longitude": "-92.84461555"
},
{
"id": 2,
"uid": null,
"name": "FAYCO",
"prefix": "FAY",
"description": "Fayette CO",
"latitude": "39.17463742",
"longitude": "-92.69534946"
},
{
"id": 3,
"uid": null,
"name": "ARMCO",
"prefix": "ARM",
"description": "Armstrong Fiber Cabinet",
"latitude": "39.26903232",
"longitude": "-92.70137640"
}
]
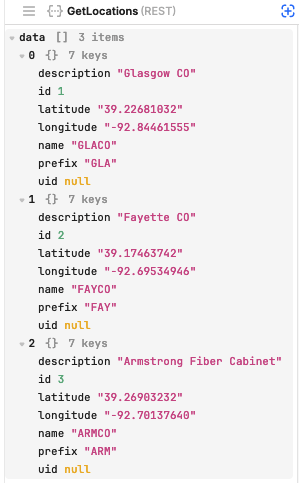
And then the second array that I am populating in a table
has a column named location_id
.
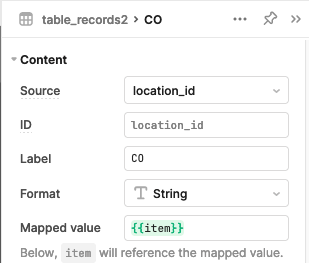
I am hoping to populate this column with the location prefix (GetLocations.data[i].prefix
), in effect, the column would say "GLA" or "FAY", depending on what the location_id is of that row. This is probably something related to .map
function, but I've did a little research and not sure how to properly formulate this expression.
Any suggestions would be appreciated!
Thanks
Two options here, depending on the size of the dataset.
For a small dataset just find
the object in getLocations.data
that contains the location_id
.
{{getLocations.data.find(x=>x.id === item).prefix}}
If your dataset is large enough that this slows table performance, I'd transform your getLocations
data into an indexed object, using the id as the key for the object.
return data.reduce((acc,obj)=>{
acc[obj.id] = obj
return acc
},{})
Now you can map the column to getLocations.data[item].prefix
and avoid having to run the code to find
the correct object each time.
Thanks!
I'm using {{GetLocations.data.find(x=>x.id === item).prefix}}
and that worked perfectly.
But I did try transforming the results of GetLocations and it worked for two of the entries, but the entry with id:1, it didn't change the key:
{
"2": {
"id": 2,
"uid": null,
"name": "FAYCO",
"prefix": "FAY",
"description": "Fayette CO",
"latitude": "39.17463742",
"longitude": "-92.69534946"
},
"3": {
"id": 3,
"uid": null,
"name": "ARMCO",
"prefix": "ARM",
"description": "Armstrong Fiber Cabinet",
"latitude": "39.26903232",
"longitude": "-92.70137640"
},
"id": 1,
"uid": null,
"name": "GLACO",
"prefix": "GLA",
"description": "Glasgow CO",
"latitude": "39.22681032",
"longitude": "-92.84461555"
}
Ah sorry, it needed a starting value of {}
in the reduce function. I edited my original reply to reflect that.
1 Like