Hi
I'm trying to map a response from an API to a table but I dont know the syntax to grab data where a Key has a specific name where I can then pull the data associated with it.
So far I've got so far -
{{item[0].scheduling[0].kvp}}
Which returns a mapped array
[[
{
"_": "My Episode Title",
"$": {
"name": "Episode Title"
}
},
{
"_": "203",
"$": {
"name": "Episode Number"
}
},
{
"_": "Season Title",
"$": {
"name": "Season Title"
}
},
{
"_": "2",
"$": {
"name": "Season Number"
}
},
{
"_": "Series",
"$": {
"name": "Program Type"
}
},
{
"_": "01:00:00:00",
"$": {
"name": "Duration"
}
}
]]
I want to pull out the values where the name matches whatever i want, for example, I want to get Episode Title and return "My Episode Title", I can get closer by running this:
{{item[0].scheduling[0].kvp[0]._}}
Which returns ["My Episode Title"] but I know that's only due to it being the first response in the array.
Thanks
you can filter the mapped array
const result = myArray.filter((arrayItem) => arrayItem.$.name === "Episode Title")
result will be an array of all items where the name was "Episode Title". if you know there will only be one match you can do something like:
const epTitle = myArray.filter((arrayItem) => arrayItem.$.name === "Episode Title")[0]._
didn't notice you have a 2d array so you might need:
let result = []
outsideArray.forEach((arrayItem) => {
result.concat(arrayItem.filter((arrayItem) => arrayItem.$.name === "Episode Title"))
}
this will check all arrays you have stored in an array (array of arrays). this would get the 'Episode Title' for every item basically
1 Like
Thanks for the response, how would I launch this filter from a table and supply is with the dataset?
im not sure where ypur data comes from, but typically youd want to make it the Transformer for a Query. I think in your case itd be easier to make a new Query and select the 'Run JS Code', you can name it filterDataByEpisode or something. then just copy+pasta the code below into the new Query.
let newData = [];
query1.data.forEach((arrayItem) => {
newData.concat(arrayItem.filter((arrayItem) => arrayItem.$.name === "Episode Title"))
}
return newData;
If you want a table to use this you would just set the data source to '{{ filterDataByEpisode.data }}'
theres a few ways to do this with the code up there, then w a ouple changes you could put it in the same spot as where you have '{{ item[0].scheduling[0].kvp }}`. another option would be to make a Success Even Handler and put this code in it but make a new variable then replace the last line with
variable1.setValue(newData);`` instead of
return newData;```. then everytime your query ran this code would also run and set the value of variable1 to whatever results it found.... in this case youd want to use {{ variable1.value }} as the datasource when you want the table to use it.
1 Like
Hi
I think I'm close, but I'm getting a .filter is not a function error.
Here's my code and the query result im interrogating:
Error
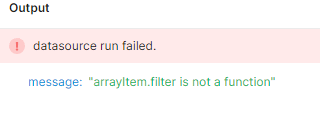
contents of arrayItem
Hey @retrofly! This is likely just a JS syntax issue. Since that data structure is a bit complex, mind sending me a bit of sample data so I can take a look?
This might be a bit of a ham-fisted approach but this is what I did to get it working -
let newData = [];
newData = getItem.data.parsedXml.item.itemmetadata[0].scheduling[0].kvp.filter(s => s.$.name === "Episode Title")[0]._
return newData
Thanks
Luke
1 Like