any way to remove values from text fields, and select etc (approx 50) all at once?
or i need to do it like this manually for each field
idcliente.clearValue();
nombres.clearValue();
apellidos.clearValue();
cedula.clearValue();
sexo.clearValue();
ubicacion.clearValue();
nivel.clearValue();
nacimiento.clearValue();
edad.clearValue();
jubilacion.clearValue();
janticipada.clearValue();
sector.clearValue();
I'm presuming the fields aren't inside a form component? That would be the usual/ideal way to control multiple related components.
Potentially you could create a variable that holds references to all the fields and loop through it - maybe easier if you're still adding fields to the app. ie you'd add the reference in one place and not have to add it to each query/event that does a clear or reset or get value function?
When you say "select" do you mean, get their values? Again, if not in a form then it's likely to involve some loop, it will depend on how many fields you have and if this is a fixed list or can be dynamic. For ease of maintenance I'd try to variable approach though.
2 Likes
A select component. Yeah i should use form but all fields have diff tables in db. I got like 5 groups so 4 fields for one table, 4 to another etc. thats why i didnt use forms
for example each tab have like 3 diff groups so they save to 3 diff tables, and so on. thats why i didnt use form as i think it will be a large app in terms of perfomance
Hello, you can do the following
const componentDir = {
"1": textInput1,
"2": textInput2,
"3": textInput3
}
for (let i = 1; i <= Object.keys(componentDir).length; i++) {
componentDir[i].clearValue()
}
Make a Dir of all your components that you want to clear its value, keeping the incremental key (1, 2, 3, 4, ...)
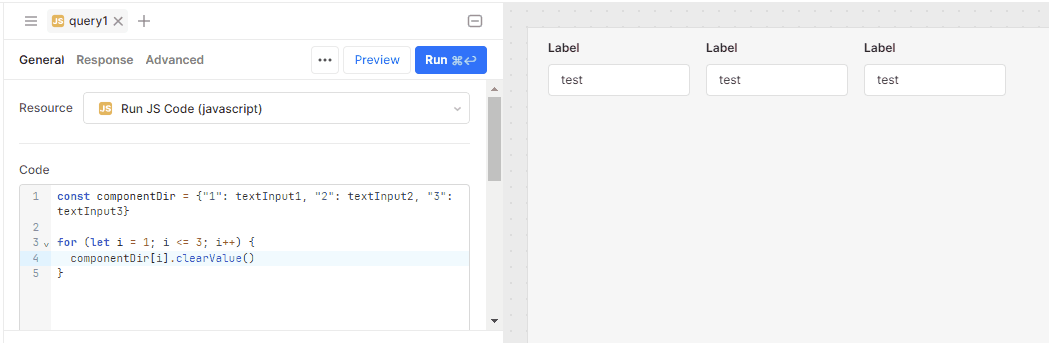
1 Like
I think @dcartlidge is a better approach:
const Array = [textInput1, textInput2, numberInput1]
for (let i = 0; i < Array.length; i++) {
Array[i].clearValue()
}
2 Likes
How far is too far? 
{{ [textInput1, textInput2, numberInput1].forEach(textInput => textInput.clearValue()) }}